Machine Learning with PySpark : Introduction to Spark MLlib | Pyspark Assignment Help
- Pushkar Nandgaonkar
- Mar 2, 2023
- 4 min read
With the advent of big data, it has become increasingly important to have scalable solutions for data processing and machine learning. PySpark is a powerful tool that allows for distributed processing of large datasets using the Apache Spark framework. One of the key components of PySpark is Spark MLlib, which provides a powerful set of tools for building and evaluating machine learning models. In this article, we will provide an overview of Spark MLlib and walk through the process of building a machine learning model using PySpark.
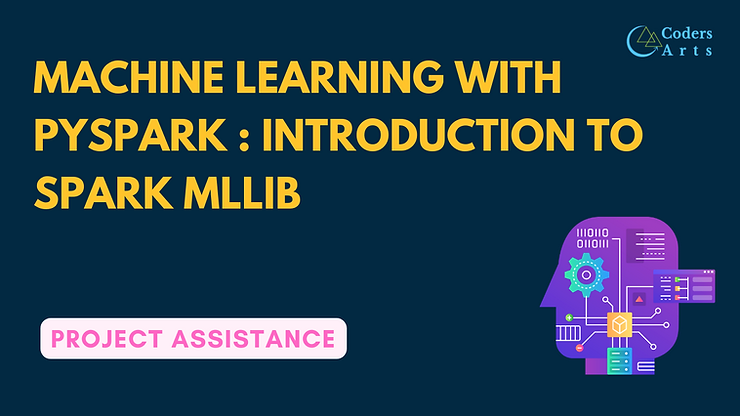
Introduction to Spark MLlib
Spark MLlib is a machine learning library built on top of Apache Spark. It provides a set of high-level APIs for common machine learning tasks such as classification, regression, clustering, and collaborative filtering. MLlib also includes tools for feature extraction, transformation, and selection.
One of the key advantages of Spark MLlib is its scalability. MLlib can efficiently handle large datasets and can be run on distributed systems, making it a popular choice for big data applications. MLlib also provides support for a wide range of machine learning algorithms, including decision trees, random forests, logistic regression, and support vector machines.
Preprocessing Data
Before building a machine learning model, it is important to preprocess the data to ensure that it is in a format suitable for machine learning algorithms. Preprocessing involves tasks such as cleaning the data, handling missing values, and transforming the data into a format that can be used by machine learning algorithms.
Spark MLlib provides a number of tools for preprocessing data. For example, the VectorAssembler class can be used to combine multiple columns into a single feature vector, which can then be used as input for machine learning algorithms. The Imputer class can be used to handle missing values by imputing values based on the mean, median, or most frequent value.
Other preprocessing tasks can include feature scaling and normalization, which can improve the performance of some machine learning algorithms. MLlib provides tools for both min-max scaling and z-score normalization.
Building Machine Learning Models
Once the data has been preprocessed, the next step is to build a machine learning model. Spark MLlib provides a wide range of machine learning algorithms, including decision trees, random forests, logistic regression, and support vector machines.
To build a machine learning model in Spark MLlib, you typically start by defining a pipeline. A pipeline is a sequence of stages, where each stage represents a transformation or a machine learning algorithm. For example, a pipeline might consist of a VectorAssembler stage, followed by a feature scaler, and then a logistic regression algorithm.
Once you have defined your pipeline, you can fit the pipeline to your data using the fit() method. This will train the machine learning model on your data and produce a trained model object.
Evaluating Models
After training a machine learning model, it is important to evaluate its performance. Spark MLlib provides a number of tools for evaluating machine learning models, including metrics such as accuracy, precision, recall, and F1-score.
To evaluate a model, you typically start by splitting your data into a training set and a test set. You then use the fit() method to train the model on the training set, and the transform() method to generate predictions on the test set. You can then use the metrics provided by MLlib to evaluate the performance of your model.
Saving and Loading Models
Once you have trained a machine learning model in Spark MLlib, you can save the model to disk for later use. This can be useful if you want to use the model in a production environment or share the model with others.
To save a model in Spark MLlib, you can use the save() method. This will save the model to a file on disk. To load a saved model, you can use the load() method.
Example: Building a Logistic Regression Model
To illustrate the process of building a machine learning model in Spark MLlib, let's walk through an example of building a logistic regression model.
Suppose we have a dataset of customer information, including features such as age, income, and credit score. We want to build a model to predict whether a customer will make a purchase or not.
The first step is to preprocess the data. We might want to handle missing values by imputing the mean value, and then use a VectorAssembler stage to combine the features into a single feature vector.
from pyspark.ml.feature import Imputer, VectorAssembler
imputer = Imputer(strategy='mean', inputCols=['age', 'income', 'credit_score'], outputCols=['age_imputed', 'income_imputed', 'credit_score_imputed'])
vector_assembler = VectorAssembler(inputCols=['age_imputed', 'income_imputed', 'credit_score_imputed'], outputCol='features')
preprocessing_pipeline = Pipeline(stages=[imputer, vector_assembler])
preprocessed_data = preprocessing_pipeline.fit(data).transform(data)
Next, we define our logistic regression model and create a pipeline that combines our preprocessing pipeline with the logistic regression algorithm.
from pyspark.ml.classification import LogisticRegression
logistic_regression = LogisticRegression(featuresCol='features', labelCol='purchased')
model_pipeline = Pipeline(stages=[preprocessing_pipeline, logistic_regression])
We can then fit the pipeline to our preprocessed data.
model = model_pipeline.fit(preprocessed_data)
Finally, we can evaluate the performance of our model on a test set.
test_data = spark.read.csv('test_data.csv', header=True, inferSchema=True)
preprocessed_test_data = preprocessing_pipeline.fit(test_data).transform(test_data)
predictions = model.transform(preprocessed_test_data)
from pyspark.ml.evaluation import BinaryClassificationEvaluator
evaluator = BinaryClassificationEvaluator(labelCol='purchased')
We can use the evaluator to compute the area under the receiver operating characteristic (ROC) curve, which is a commonly used metric for binary classification problems.
roc_auc = evaluator.evaluate(predictions)
print('ROC AUC:', roc_auc)
Saving and Loading Models
If we are happy with the performance of our model, we might want to save it to disk for later use. We can do this using the save() method.
model.save('logistic_regression_model')
To load the saved model, we can use the load() method.
loaded_model = PipelineModel.load('logistic_regression_model')
Conclusion
In this article, we have introduced the basics of using PySpark and Spark MLlib for machine learning. We have shown how to preprocess data, build machine learning models, evaluate models, and save and load models for later use.
Spark MLlib provides a wide range of machine learning algorithms and tools for working with large datasets, making it a powerful tool for data scientists and machine learning engineers. By leveraging the distributed computing capabilities of Spark, we can train machine learning models on massive datasets that would be too large to fit into memory on a single machine.
While the examples in this article have focused on binary classification problems, Spark MLlib also supports a wide range of other machine learning tasks, including regression, clustering, and recommendation systems. With its powerful distributed computing capabilities and rich set of algorithms, Spark MLlib is a powerful tool for data scientists and machine learning engineers working on large-scale machine learning projects.

Comments