Introduction
In Android app development, it's common to fetch data from APIs and store it locally for offline access or to minimize network calls. In this tutorial, we'll explore how to make API calls using Retrofit and store the response locally using PaperDB, a simple NoSQL database for Android. We'll also demonstrate how to handle API calls only once a day or when data is not available in the local database.
Prerequisites
To follow along with this tutorial, make sure you have the following:
Android Studio installed
Basic understanding of Android app development
Familiarity with Kotlin programming language

Setting up the Project
Create a new Android project in Android Studio with an appropriate name (e.g., "ApiCallProject").
Add the necessary dependencies in the app-level build.gradle file:
// Retrofit dependencies
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
implementation 'com.squareup.retrofit2:adapter-rxjava2:2.9.0'
// RxJava dependencies
implementation 'io.reactivex.rxjava2:rxjava:2.2.21'
implementation 'io.reactivex.rxjava2:rxandroid:2.1.1'
// PaperDB dependency
implementation 'io.paperdb:paperdb:2.7'
3. Sync the project to download the added dependencies.
Creating the Data Models
To represent the API response and the corresponding data models, create the following Kotlin data classes:
data class ApiResponse(
val facilities: List<Facility>,
val exclusions: List<List<Exclusion>>
)
data class Facility(
val facilityId: String,
val name: String,
val options: List<FacilityOption>
)
data class FacilityOption(
val name: String,
val icon: String,
val id: String
)
data class Exclusion(
val facilityId: String,
val optionsId: String
)
Making API Calls with Retrofit
To make API calls, we'll use Retrofit, a popular HTTP client library for Android. Retrofit simplifies the process of sending network requests and parsing the responses. Follow these steps to integrate Retrofit into your project:
Create an interface ApiService to define the API endpoints:
interface ApiService {
@GET("api/facilities")fun getFacilities(): Single<ApiResponse>
}
Using the Retrieved Data
To display the retrieved facilities in the UI, we'll assume that you have a RecyclerView and a custom adapter. Here's an example of how you can use the retrieved facilities in the activity or fragment:
Create an adapter class, FacilityAdapter, to bind the facility data to the RecyclerView:
class FacilityAdapter(
private val facilities: List<Facility>,
private val context: Context
) : RecyclerView.Adapter<FacilityAdapter.FacilityViewHolder>() {
// ViewHolder implementation goes hereoverride fun getItemCount(): Int {
return facilities.size
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): FacilityViewHolder {
// Inflate the item layout and return a new ViewHolderval view = LayoutInflater.from(context).inflate(R.layout.item_facility, parent, false)
return FacilityViewHolder(view)
}
override fun onBindViewHolder(holder: FacilityViewHolder, position: Int) {
// Bind the facility data to the ViewHolderval facility = facilities[position]
holder.bind(facility)
}
inner class FacilityViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
// ViewHolder implementation goes herefun bind(facility: Facility) {
// Bind the facility data to the views
itemView.facilityNameTextView.text = facility.name
// Set other views accordingly
}
}
}
In the activity or fragment where you want to make the API call, add the following method to fetch the facilities:
private fun getFacilities(): Single<ApiResponse> {
val retrofit = Retrofit.Builder()
.baseUrl("https://my-json-server.typicode.com/")
.addConverterFactory(GsonConverterFactory.create())
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
val apiService = retrofit.create(ApiService::class.java)
return apiService.getFacilities()
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
}
Note: Ensure that you have added the necessary imports for Retrofit and RxJava.
Storing API Response Locally with PaperDB
PaperDB is a lightweight NoSQL database for Android that simplifies local storage operations. Follow these steps to integrate PaperDB into your project:
Initialize PaperDB in the onCreate method of your application class or the activity where you want to use it:
Paper.init(applicationContext)
To store the API response in PaperDB, modify the getFacilities method as follows:
private fun getFacilities(): Single<ApiResponse> {
val storedApiResponse: ApiResponse? = Paper.book().read("apiResponse")
if (storedApiResponse != null) {
// Return the stored data if available
return Single.just(storedApiResponse)
} else {
// Fetch the facilities from the API
val retrofit = Retrofit.Builder()
.baseUrl("https://my-json-server.typicode.com/")
.addConverterFactory(GsonConverterFactory.create())
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
val apiService = retrofit.create(ApiService::class.java)
return apiService.getFacilities()
.doOnSuccess { response ->
// Save the response to PaperDB
Paper.book().write("apiResponse", response)
}
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
}
}
Note: Ensure that you have added the necessary import for PaperDB.
Using the Retrieved Data
To display the retrieved facilities in the UI, we'll assume that you have a RecyclerView and a custom adapter. Here's an example of how you can use the retrieved facilities in the activity or fragment:
private fun showFacilities(facilities: List<Facility>) {
val adapter = FacilityAdapter(facilities, this)
recyclerView.adapter = adapter
}
Output:
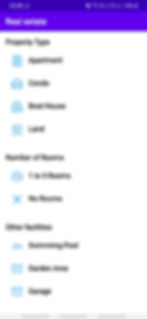
Conclusion
In this tutorial, we've learned how to make API calls using Retrofit and store the response locally using PaperDB in an Android app. We've also implemented a mechanism to fetch data from the API only once a day or when the data is not available in the local database. By combining these techniques, you can ensure efficient data retrieval and provide a seamless user experience in your app.
I hope this tutorial was helpful in understanding API calls and local storage with PaperDB in Android. Feel free to explore more features of Retrofit and PaperDB to enhance your app's functionality.
Whether you need assistance with Android app development, UI/UX design, debugging, or any other aspect of Android programming, our experts are ready to provide you with tailored solutions. We cover a wide range of topics, including Android architecture, Kotlin programming, database integration, API integration, and more.
Why choose CodersArts?
Expert Assistance: Our team consists of highly skilled Android developers who have extensive experience in the field. They are proficient in the latest tools, frameworks, and technologies, ensuring top-notch solutions for your assignments.
Timely Delivery: We understand the importance of deadlines. Our experts work diligently to deliver your completed assignments on time, allowing you to submit your work without any worries.
Customized Solutions: We believe in providing personalized solutions that meet your specific requirements. Our experts carefully analyze your assignment and provide tailored solutions to ensure your success.
Affordable Pricing: We offer competitive and affordable pricing to make our services accessible to students. We understand the financial constraints that students often face, and our pricing is designed to accommodate their needs.
24/7 Support: Our customer care team is available round the clock to assist you with any queries or concerns you may have. You can reach us via phone, email, or live chat.
Contact CodersArts today for reliable and professional Android assignment help. Let our experts take your Android programming skills to the next level!
