Introduction
In the world of computer science and programming, data structure plays an important role in organizing and manipulating data efficiently. One of the data structure is stack. Stacks are not only a fundamental concept in computer science but also a practical tool for solving a wide range of problems. In Java, stacks follow the Last-In-First-Out (LIFO) principle. In this blog, we are going to see the stack class, their methods and their use. Also, the practical example where we use a stack.

Understanding
Definition: The stack is a linear data structure that is used to store the collection of objects. It is based on Last-In-First-Out (LIFO).
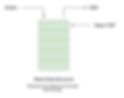
Types:
Fixed-Size stack: A fixed-size stack as the name suggests has a predefined size that we can't change after the creation. It has implemented an array of fixed length.
Dynamic stack: A dynamic stack is also known as a resizable stack to overcome the limitation of fixed size allowing to change size at runtime.
Methods:
Push(Element): This method is used to insert the element in the stack.
Pop(): This method is used to get the top element and remove the element from the stack.
Peek(): This method is used to get the top element without removing the element.
Search(Element): This method is used to check whether the element is present or not. If an element is present then it will return the index if not then it will return the -1.
Practical Example:
Web Browser History: Web browsers use stacks to implement the "Back" and "Forward" functionality. When you navigate from one web page to another, the visited URLs are pushed onto a stack. Pressing the "Back" button pops the top URL from the stack, allowing you to revisit previously visited pages.
Text Editor Undo/Redo Functionality: Text editors often provide undo and redo functionalities to revert or reapply changes made to a document. A stack can be used to implement these functionalities. Each time a change is made, the modified text or action is pushed onto the stack. When the user clicks the "Undo" button, the most recent change is popped from the stack and reverted.
Implementation
import java.util.Stack;
public class Demo {
public static void main(String[] args) {
Stack<String> stack = new Stack<String>();
// To add the elements in stack
stack.push("Java");
stack.push("Python");
stack.push("C++");
// To print the stack
System.out.println("Stack: " + stack);
// To Remove element and to get top element
String topElement = stack.pop();
System.out.println("Popped element: " + topElement);
System.out.println("Stack after pop: " + stack);
// To get the element without removing element
String peekElement = stack.peek();
System.out.println("Peeked element: " + peekElement);
System.out.println("Stack after peek: " + stack);
}
}
At CodersArts
Expert Assistance: Our team consists of highly skilled Java, and Spring Boot developers who have extensive experience in the field. They are proficient in the latest tools, frameworks, and technologies, ensuring top-notch solutions for your assignments, Projects.
Timely Delivery: We understand the importance of deadlines. Our experts work diligently to deliver your completed assignments, Projects on time, allowing you to submit your work without any worries.
Customized Solutions: We believe in providing personalized solutions that meet your specific requirements. Our experts carefully analyze your assignment or Project and provide tailored solutions to ensure your success.
Affordable Pricing: We offer competitive and affordable pricing to make our services accessible to students, Developers. We understand the financial constraints that students, Developers often face, and our pricing is designed to accommodate their needs.
24/7 Support: Our customer care team is available round the clock to assist you with any queries or concerns you may have. You can reach us via phone, email, or live chat.
Contact CodersArts today for reliable and professional Spring Boot, Spring Security, and Java help. Let our experts take your Java programming skills to the next level!
