Queue-nique: Unleashing the Power of Queues in Java
- Swapnil V
- Jul 14, 2023
- 3 min read
Introduction
Queue is a fundamental data structure that holds a special place in the world of programming. They provide an effective manner to manipulate data, facilitate communication, and cure complicated troubles efficiently. Queue in Java is a linear data structure where you can handle the ordered collection of elements. It follows the FIFO principle to add elements from one end and remove them from the other end. In this blog, we are going to see the implementation and use of methods that are present in the queue.
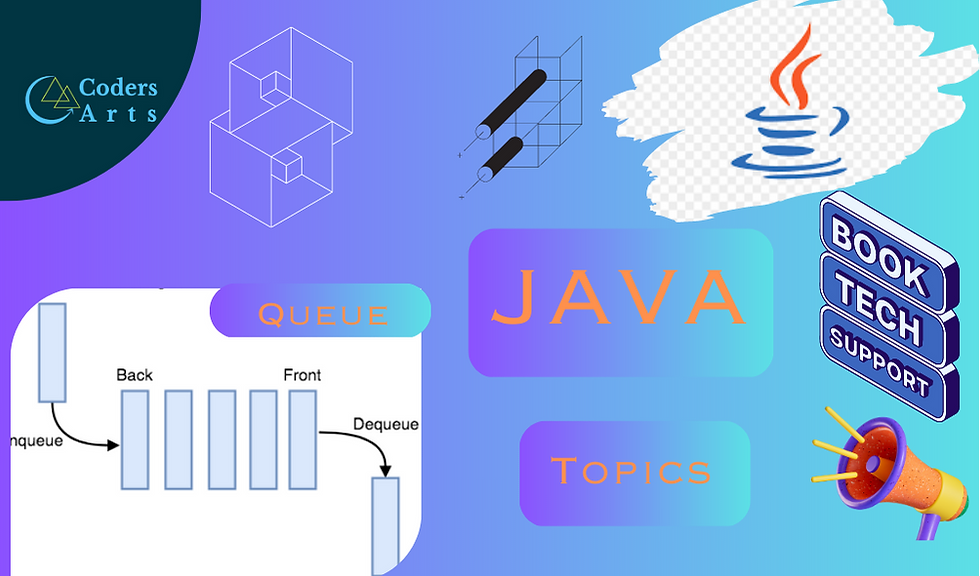
Understanding
Definition: A queue is a linear data structure that consists of a collection of items that follow a first-in-first-out sequence. This implies that the first item to be inserted will be the first to be removed.
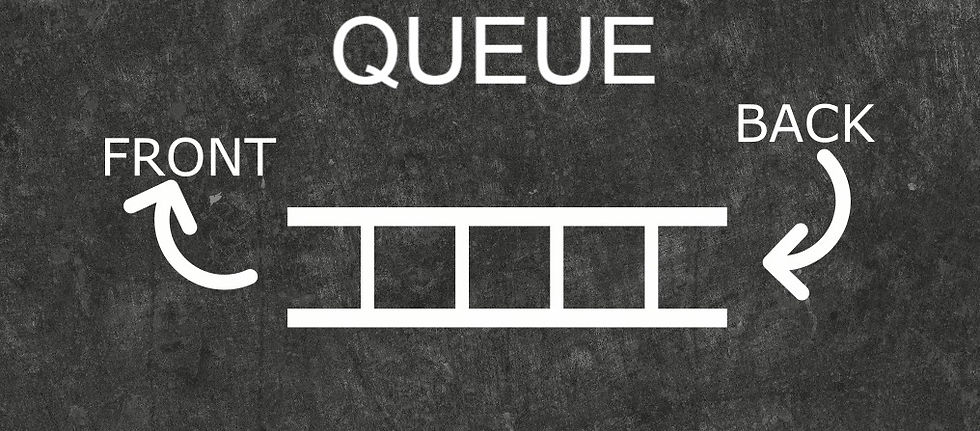
Types:
PriorityQueue: PriorityQueue is a class that is defined in the collection framework that gives us a way for processing the objects on the basis of priority.
PriorityQueue<DataType> priorityQueue = new PriorityQueue<DataType>();
LinkedList: LinkedList is a class which is implemented in the collection framework which implements the linked list data structure. It is a linear data structure where the elements are not stored in contiguous locations and every element is a separate object with a data part and address part.
LinkedList<DataType> linkedlist= new LinkedList<DataType>();
ArrayDeque: The ArrayDeque in Java provides a way to apply resizable arrays in addition to the implementation of the Deque interface. It is also known as Array Double Ended Queue or Array Deck.
ArrayDeque<DataType> arrayDeque= new ArrayDeque<DataType>();
Methods
Add: Adds the specified element to the queue. Returns "true" if the element was successfully added, otherwise throws an exception. Eg.IllegelStateException or NullPointerException.
Offer: Adds the specified element to the queue. Returns "true" if the element was successfully added, otherwise returns "false".
Element: Return, but does not remove, the element at the front of the queue. Throws an exception (e.g., NoSuchElementException) if the queue is empty.
Peek: Return, but does not remove, the element at the front of the queue. Returns null if the queue is empty.
Remove: Return and removes the element at the front of the queue. Throws an exception (e.g., NoSuchElementException) if the queue is empty.
Poll: Return and removes the element at the front of the queue. Returns null if the queue is empty.
import java.util.LinkedList;
import java.util.Queue;
public class Demo {
public static void main(String[] args) {
Queue<String> queue = new LinkedList<>();
// Adding methods in the queue
queue.add("A");
queue.add("B");
queue.add("C");
queue.offer("D");
System.out.println("Queue: " + queue);
// Getting front elements in the queue
String frontElement = queue.element();
System.out.println("Front element: " + frontElement);
String peekElement = queue.peek();
System.out.println("Peek element: " + peekElement);
// Remove elements from the queue
String removeElement = queue.remove();
System.out.println("Remove element: " + removeElement);
String pollElement = queue.poll();
System.out.println("Poll element: " + pollElement);
System.out.println("Queue after: " + queue);
}
}
At CodersArts
Expert Assistance: Our team consists of highly skilled Java, and Spring Boot developers who have extensive experience in the field. They are proficient in the latest tools, frameworks, and technologies, ensuring top-notch solutions for your assignments, Projects.
Timely Delivery: We understand the importance of deadlines. Our experts work diligently to deliver your completed assignments, Projects on time, allowing you to submit your work without any worries.
Customized Solutions: We believe in providing personalized solutions that meet your specific requirements. Our experts carefully analyze your assignment or Project and provide tailored solutions to ensure your success.
Affordable Pricing: We offer competitive and affordable pricing to make our services accessible to students, Developers. We understand the financial constraints that students, Developers often face, and our pricing is designed to accommodate their needs.
24/7 Support: Our customer care team is available round the clock to assist you with any queries or concerns you may have. You can reach us via phone, email, or live chat.
Contact CodersArts today for reliable and professional Spring Boot, Spring Security, and Java help. Let our experts take your Java programming skills to the next level!

Σχόλια