Programming in R: A Comprehensive Guide | R Programming Assignment Help
- Pushkar Nandgaonkar
- Mar 2, 2023
- 3 min read
R is a popular programming language used in data science, statistical computing, and data analysis. It offers a wide range of tools and functions for data manipulation, visualization, and modeling. In this article, we will discuss some of the essential concepts in programming in R, including control structures, functions, debugging and error handling, and object-oriented programming.
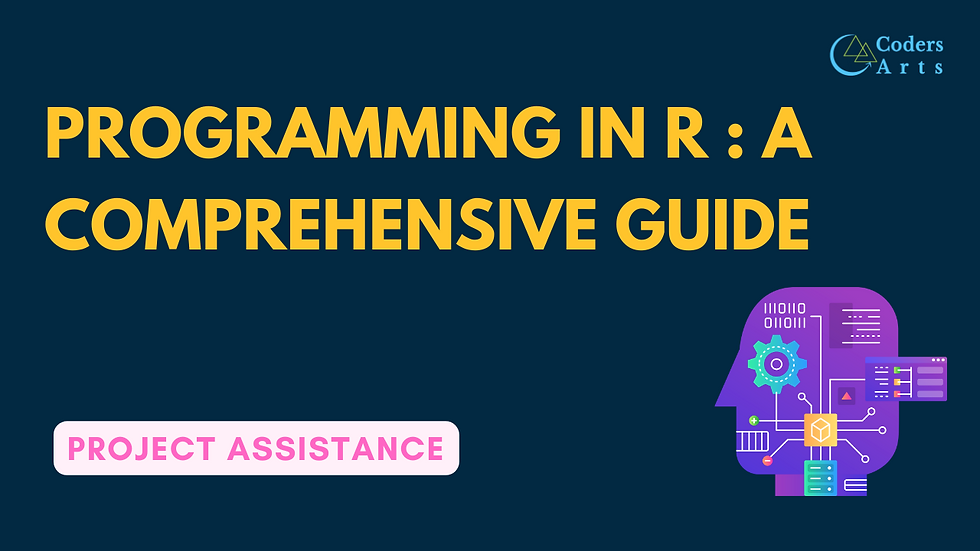
Control structures in R
Control structures are statements that allow you to control the flow of execution of a program. In R, there are three main types of control structures:
Conditional statements: Conditional statements are used to test whether a condition is true or false and then execute a particular set of statements based on the result. The most commonly used conditional statement in R is the if-else statement. The if-else statement takes a logical expression and executes a set of statements if the expression is true and a different set of statements if the expression is false.
For example:
x <- 5
if (x > 0) {
print("x is positive")} else {
print("x is non-positive")}
Looping statements: Looping statements are used to repeat a set of statements multiple times. The most commonly used looping statement in R is the for loop. The for loop takes a variable, a sequence of values, and a set of statements and executes the statements for each value of the variable.
For example:
for (i in 1:5) {
print(i)}
Control flow statements: Control flow statements are used to change the flow of execution of a program. The most commonly used control flow statement in R is the break statement, which is used to terminate a loop prematurely.
For example:
for (i in 1:10) {if (i == 5) {break}
print(i)}
Functions in R
Functions are blocks of code that perform a specific task. In R, functions are defined using the function() keyword. A function can take one or more arguments and can return a value.
For example:
# Define a function to calculate the factorial of a number
factorial <- function(n) {if (n == 0) {return(1)} else {return(n * factorial(n - 1))}}# Call the function to calculate the factorial of 5
factorial(5)
Debugging and error handling in R
Debugging is the process of finding and fixing errors in a program. In R, there are several tools and techniques for debugging, including:
The traceback() function: The traceback() function is used to print a stack trace of the function call that led to an error.
For example:
# Define a function with an error
myfunction <- function(x) {
y <- x + 1
z <- y / 0return(z)}# Call the function to generate an error
myfunction(5)
Output:
Error in myfunction(5) :
Fehler in z/y : Durch Null geteilt
Ruft auf: myfunction(5)
The debug() function: The debug() function is used to set a breakpoint in a function and enter debugging mode when the breakpoint is reached.
For example:
# Define a function with a breakpoint
myfunction <- function(x) {
y <- x + 1
debug()
z <- y / 0return(z)}# Call the function to enter debugging mode
myfunction(5)
The tryCatch() function: The tryCatch() function is used to handle errors and exceptions in R programs. It takes a set of statements to be executed and a set of handlers to be executed in case of an error or exception.
For example:
# Define a function with an error
myfunction <- function(x) {
y <- x + 1
z <- y / 0return(z)}# Use tryCatch() to handle the error
result <- tryCatch(myfunction(5), error = function(e) {
print("An error occurred")
print(e)})# Print the result
print(result)
Object-oriented programming in R
Object-oriented programming (OOP) is a programming paradigm that uses objects to represent real-world entities and their interactions. R supports OOP through the S3, S4, and reference classes systems.
In the S3 system, objects are represented as lists with class attributes. Methods are defined as functions that take an object as their first argument and are dispatched based on the class of the object.
For example:
# Define a class
setClass("person", representation(name = "character", age = "numeric"))# Define a method
print.person <- function(x) {
cat("Name:", x@name, "\n")
cat("Age:", x@age, "\n")}# Create an object
person1 <- new("person", name = "John Doe", age = 30)# Call the method
print(person1)
Conclusion
Programming in R is an essential skill for data scientists and statisticians. In this article, we have covered some of the essential concepts in programming in R, including control structures, functions, debugging and error handling, and object-oriented programming. By mastering these concepts, you can write efficient and reliable code for data manipulation, visualization, and modeling in R.

Commentaires