In this blog we will see how to build a simple blog web application using Django. The home page will list all blog posts. The aim of this blog will show you how to create a simple blog website using Django. So let's start.
In my previous blog of Django we have seen about the django framework. If you are not aware about Django you can check out my Django blog. Click here
Step 1
Create an empty folder, set path, and Now run the following command in command prompt to create a Django project

Step 2
Set path and create virtual environment for the django project by using the follwing commands as shown in the screenshot.

Step 2
Install all dependencies in the virtual environment needed for the django project using pip. Here we install django library.

Step 3
Navigate the directory where manage.py script exists and run the below command.
python manage.py startapp App

After running the above command one folder created in the my_blog_site folder.


Step 3
Add 'App' in INSTALLED_APPS
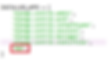
Edit model.py file located Blog_site-->my_blog_site-->App
from django.db import models
from django.contrib.auth.models import User
# Create your models here.
STATUS = ((0, "Draft"), (1, "Published"))
class Post(models.Model):
title = models.CharField(max_length=200, unique=True)
slug = models.SlugField(max_length=200, unique=True)
author = models.ForeignKey(User, on_delete=models.CASCADE, related_name='blog_posts')
created_on = models.DateTimeField(auto_now_add=True)
updated_on = models.DateTimeField(auto_now=True)
content = models.TextField()
status = models.IntegerField(choices=STATUS, default=0)
class Meta:
ordering = ['-created_on']
def __str__(self):
return self.title
Now make migrations. run the following command
python manage.py migrations

After successfully migrations. run
python manage.py migrate
This will apply all the unapplied migrations on the SQLite database which comes along with the Django installation.

Lets test our configurations by running the django project.
python manage.py runserver
open your browser and go the address http://127.0.0.1:8000/. we can see there is no error, our project is running successfully.

Create a admin site.
Here we create a admin panel to manage post for that we need to create superuser by running the follwing command.
python manage.py createsuperuser
You can enter any details for admin details.

Now run the project
python manage.py runserver
if you Goto this address : http://127.0.0.1:8000/admin/ you will this login page

After successfull login you will access the admin panel but we cant yet add post bcacuse we will need to register our post class inside our admin file.

Register post class
from django.contrib import admin
# Register your models here.
from .models import Post
class PostAdmin(admin.ModelAdmin):
list_display = ('title', 'slug', 'status', 'created_on')
list_filter = ('status',)
search_fields = ['title', 'content']
admin.site.register(Post, PostAdmin)
How Codersarts can Help you in Django ?
Codersarts provide:
Django Assignment help
Django Error Resolving Help
Mentorship in Django from Experts
Dajngo Development Project
If you are looking for any kind of Help in Django Contact us