Basics Syntax of R Programming | R Programming Assignment Help
- Pushkar Nandgaonkar
- Mar 2, 2023
- 4 min read
Introduction
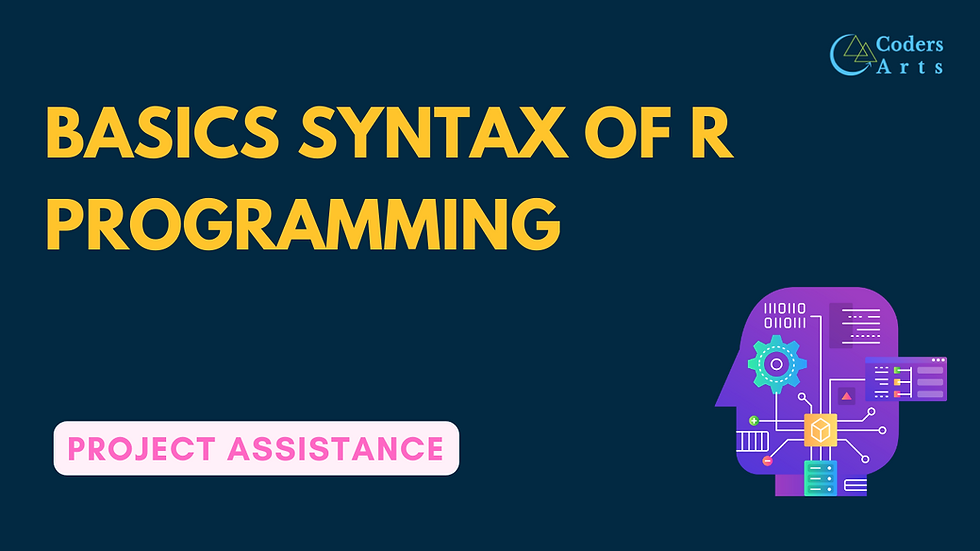
R is a popular programming language used for data analysis, statistical computing, and graphical representation. It is widely used in various industries such as healthcare, finance, and marketing. R programming syntax is easy to learn, and it is free and open-source software. This article aims to provide an overview of the basic R programming syntax, including variables and data types, vectors and matrices, arrays and data frames, and operators in R.
Variables and Data Types In R, variables are used to store data values. A variable name is a combination of letters, numbers, and underscores. Variable names are case-sensitive. To assign a value to a variable, the assignment operator "<-" or "=" is used. For example,
x <- 10
y = 20
In the above example, the value 10 is assigned to variable x, and the value 20 is assigned to variable y.
R has various data types, including numeric, character, logical, and complex. Numeric data type represents numbers, and it can be either integers or floating-point numbers. Character data type represents text, and it is enclosed in quotes. Logical data type represents true or false values. Complex data type represents complex numbers, which are numbers with a real and imaginary part.
To determine the data type of a variable, the class() function is used.
For example,
x <- 10
class(x)# output: "numeric"
y <- "Hello, world!"class(y)# output: "character"
z <- TRUEclass(z)# output: "logical"c <- 2 + 3iclass(c)# output: "complex"
Vectors and Matrices In R, a vector is a collection of elements of the same data type. Vectors can be created using the c() function.
For example,
v1 <- c(1, 2, 3, 4, 5)
v2 <- c("apple", "banana", "cherry")
v3 <- c(TRUE, FALSE, TRUE)
In the above example, v1 is a numeric vector, v2 is a character vector, and v3 is a logical vector.
R also has a matrix data structure, which is a two-dimensional array. Matrices can be created using the matrix() function.
For example,
m1 <- matrix(c(1, 2, 3, 4, 5, 6), nrow = 2, ncol = 3)
m2 <- matrix(c("red", "green", "blue"), nrow = 1, ncol = 3)
In the above example, m1 is a numeric matrix with two rows and three columns, and m2 is a character matrix with one row and three columns.
Arrays and Data Frames R has an array data structure, which is a multi-dimensional extension of a matrix. Arrays can be created using the array() function.
For example,
a1 <- array(c(1, 2, 3, 4, 5, 6), dim = c(2, 3, 2))
a2 <- array(c("red", "green", "blue", "yellow"), dim = c(2, 2, 1))
In the above example, a1 is a numeric array with two rows, three columns, and two layers, and a2 is a character array with two rows, two columns, and one layer.
Another important data structure in R is the data frame, which is a two-dimensional table-like structure. Data frames can be created using the data.frame() function. For example,
df <- data.frame(name = c("John", "Jane), age = c(25, 30), gender = c("male", "female"))
In the above example, df is a data frame with three variables: name, age, and gender, and two observations. Operators in R R has various operators that can be used to perform mathematical and logical operations on variables and data structures. Some of the commonly used operators in R are: - Arithmetic operators: +, -, *, /, ^, %/%, %* Arithmetic operators are used to perform mathematical operations such as addition, subtraction, multiplication, division, and exponentiation. The %/% operator returns the integer quotient, and the %% operator returns the remainder.
a <- 10
b <- 5
c <- a + b
d <- a - b
e <- a * b
f <- a / b
g <- a^b
h <- a %/% b
i <- a %% b
output: 15, 5, 50, 2, 100000, 2, 0
Comparison operators: <, >, <=, >=, ==, !=
Comparison operators are used to compare two valuesandreturn a logical value (TRUEorFALSE).
a <- 10
b <- 5
c <- a > b
d <- a <= b
e <- a == b
f <- a != b
output: TRUE, TRUE, FALSE, TRUE
Logical operators: !, &, | Logical operators are used to perform logical operations on logical values. The ! operator returns the negation of a logical value. The & operator returns TRUE only if both operands are TRUE. The | operator returns TRUE if at least one operand is TRUE.
a <- TRUE
b <- FALSE
c <- !a
d <- a & b
e <- a | b
output: FALSE, FALSE, TRUE
Assignment operators: <-, = Assignment operators are used to assign a value to a variable.
a <- 10
b = 5
output: 10, 5
Conclusion
In conclusion, R is a powerful programming language used for data analysis, statistical computing, and graphical representation. R programming syntax is easy to learn and understand, and it has various data types and data structures that can be used to store and manipulate data. Variables and data types are used to store and represent data values, while vectors and matrices, arrays, and data frames are used to store and manipulate collections of data. R also has various operators that can be used to perform mathematical and logical operations on variables and data structures. Understanding the basic R programming syntax is essential for anyone who wants to learn and use R for data analysis and statistical computing.

Comments