Microservices :
It is an architectural style that structures an application as a collection of services that are
Highly maintainable and testable
Loosely coupled
Independently deployable
Organized around business capabilities
Owned by a small team
The microservice architecture enables the rapid, frequent and reliable delivery of large, complex applications. It also enables an organization to evolve its technology stack with Spring Boot, your microservices can start small and iterate fast. That’s why it has become the de facto standard for Java™ microservices.
How Does Microservice Architecture Work?
A typical Microservice Architecture (MSA) should consist of the following components:
Clients
Identity Providers
API Gateway
Messaging Formats
Databases
Static Content
Management
Service Discovery
1. Clients
The architecture starts with different types of clients, from different devices trying to perform various management capabilities such as search, build, configure etc.
2. Identity Providers
These requests from the clients are then passed on the identity providers who authenticate the requests of clients and communicate the requests to API Gateway. The requests are then communicated to the internal services via well-defined API Gateway.
3. API Gateway
Since clients don’t call the services directly, API Gateway acts as an entry point for the clients to forward requests to appropriate microservices.
The advantages of using an API gateway include:
All the services can be updated without the clients knowing.
Services can also use messaging protocols that are not web-friendly.
The API Gateway can perform cross-cutting functions such as providing security, load balancing etc.
After receiving the requests of clients, the internal architecture consists of microservices which communicate with each other through messages to handle client requests.
4. Messaging Formats
There are two types of messages through which they communicate:
Synchronous Messages: In the situation where clients wait for the responses from a service, Microservices usually tend to use REST (Representational State Transfer) as it relies on a stateless, client-server, and the HTTP protocol. This protocol is used as it is a distributed environment each and every functionality is represented with a resource to carry out operations
Asynchronous Messages: In the situation where clients do not wait for the responses from a service, Microservices usually tend to use protocols such as AMQP, STOMP, MQTT. These protocols are used in this type of communication since the nature of messages is defined and these messages have to be interoperable between implementations.
5. Data Handling
Well, each Microservice owns a private database to capture their data and implement the respective business functionality. Also, the databases of Microservices are updated through their service API only
6. Static Content
After the Microservices communicate within themselves, they deploy the static content to a cloud-based storage service that can deliver them directly to the clients via Content Delivery Networks (CDNs).
Apart from the above components, there are some other components appear in a typical Microservices Architecture:
7. Management
This component is responsible for balancing the services on nodes and identifying failures.
8. Service Discovery
Acts as a guide to Microservices to find the route of communication between them as it maintains a list of services on which nodes are located.
Advantage of Microservices :
The smaller code base is easy to maintain.
Easy to scale as an individual component.
Technology diversity i.e. we can mix libraries, databases, frameworks etc.
Fault isolation i.e. a process failure should not bring the whole system down.
Better support for smaller and parallel team.
Independent deployment
Deployment time reduce
To build a simple microservices using SpringBoot system following steps required
Creating Discovery Service (Creating Eureka Discovery Service)
Creating MicroService (the Producer)
Register itself with Discovery Service with logical service.
Create Microservice Consumers find Service registered with Discovery Service
Discovery client using a smart RestTemplate to find microservice.
Maven Dependencies
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.hsqldb</groupId>
<artifactId>hsqldb</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Step 1: Creating Discovery Service (Creating Eureka Discovery Service)
Eureka Server using Spring Cloud
We need to implement our own registry service as below.
application.yml
# Configure this Discovery Server
eureka:
instance:
hostname: localhost
client: #Not a client
registerWithEureka: false
fetchRegistry: false
# HTTP (Tomcat) port
server:
port: 1111
DiscoveryMicroserviceServerApplication.java
package com.doj.discovery;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class DiscoveryMicroserviceServerApplication {
public static void main(String[] args) {
SpringApplication.run(DiscoveryMicroserviceServerApplication.class, args);
}
}
pom.xml
<!-- Eureka registration server -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka-server</artifactId>
</dependency>
For Whole Source Code for the Discover Server Application, you could download from GitHub as below link.
Run this Eureka Server application with right click and run as Spring Boot Application and open in browser http://localhost:1111/

Step 2: Creating Account Producer MicroService Microservice declares itself as an available service and register to Discovery Server created in Step 1.
Advertisements
Using @EnableDiscoveryClient
Registers using its application name
Let’s see the service producer application structure as below.
application.yml
### Spring properties
# Service registers under this name
spring:
application:
name: accounts-microservice
# Discovery Server Access
eureka:
client:
serviceUrl:
defaultZone: http://localhost:1111/eureka/
# HTTP Server (Tomcat) Port
server:
port: 2222
# Disable Spring Boot's "Whitelabel" default error page, so we can use our own
error:
whitelabel:
enabled: false
AccountsMicroserviceServerApplication.java
package com.doj.ms.accounts;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
@EnableDiscoveryClient
public class AccountsMicroserviceServerApplication {
public static void main(String[] args) {
SpringApplication.run(AccountsMicroserviceServerApplication.class, args);
}
}
pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Other required source files related to this application you could download from GitHub link as given below
accounts-microservice-server
Now run this account service application as Spring Boot application and after few seconds refresh the browser to the home page of Eureka Discovery Server at http://localhost:1111/
Step 1. Now one Service registered to the Eureka registered instances with Service Name “ACCOUNT-MICROSERVICE” as below

Let’s see the consumer application structure as below.
application.yml
# Service registers under this name
# Control the InternalResourceViewResolver:
spring:
application:
name: accounts-web
mvc:
view:
prefix: /WEB-INF/views/
suffix: .jsp
# Discovery Server Access
eureka:
client:
serviceUrl:
defaultZone: http://localhost:1111/eureka/
# Disable Spring Boot's "Whitelabel" default error page, so we can use our own
error:
whitelabel:
enabled: false
WebclientMicroserviceServerApplication.java
package com.doj.web;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
@EnableDiscoveryClient
public class WebclientMicroserviceServerApplication {
public static final String ACCOUNTS_SERVICE_URL = "http://ACCOUNTS-MICROSERVICE";
public static void main(String[] args) {
SpringApplication.run(WebclientMicroserviceServerApplication.class, args);
}
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
@Bean
public AccountRepository accountRepository(){
return new RemoteAccountRepository(ACCOUNTS_SERVICE_URL);
}
}
pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-ribbon</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- These dependencies enable JSP usage -->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
</dependency>
</dependencies>
Other required source files related to this application you could download from GitHub link as given below
Now run this consumer service application as Spring Boot application and after few seconds refresh the browser to the home page of Eureka Discovery Server at http://localhost:1111/ in previous
Step 1. Now one more Service registered to the Eureka registered instances with Service Name “ACCOUNTS-WEB” as below

Lets our consumer consume the service of producer registered at discovery server.
package com.doj.web;
import java.util.Arrays;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.client.RestTemplate;
public class RemoteAccountRepository implements AccountRepository {
@Autowired
protected RestTemplate restTemplate;
protected String serviceUrl;
public RemoteAccountRepository(String serviceUrl) {
this.serviceUrl = serviceUrl.startsWith("http") ? serviceUrl
: "http://" + serviceUrl;
}
@Override
public List<Account> getAllAccounts() {
Account[] accounts = restTemplate.getForObject(serviceUrl+"/accounts", Account[].class);
return Arrays.asList(accounts);
}
@Override
public Account getAccount(String number) {
return restTemplate.getForObject(serviceUrl + "/accounts/{id}",
Account.class, number);
}
}
Let’s open web application which is a consumer of the account microservice registered at Eureka Discovery Server.
http://localhost:8080/ as below

Now click on View Account List then fetch all accounts from account microservice.
http://localhost:8080/accountList

Now click on any account from the list of accounts to fetch the details of the account for account number from account microservice.
http://localhost:8080/accountDetails?number=5115
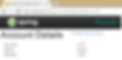
Load Balanced RestTemplate Create using @LoadBalanced– Spring enhances it to service lookup & load balancing
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
Must inject using the same qualifier-
If there are multiple RestTemplate you get the right one.
It can be used to access multiple microservices
@Autowired
@LoadBalanced
protected RestTemplate restTemplate;
Load Balancing with Ribbon Our smart RestTemplate automatically integrates two Netflix utilities
Eureka Service Discovery
Ribbon Client Side Load Balancer
Eureka returns the URL of all available instances Ribbon determine the best available service too use
Just inject the load balanced RestTemplate automatic lookup by logical service-name
How does CodersArts helps you ?
CodersArts provide :
Spring boot microservices assignment Help
Help in springboot development Projects
Mentorship from Experts Live 1:1 session
Course and Project completions
CourseWork help