Sending Email - Spring Boot Project Sample
- praveen3197
- Nov 5, 2020
- 2 min read
Updated: Jan 29, 2021
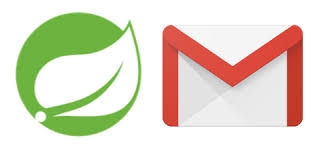
In this article, we will learn step by step to send emails using Spring Boot Application. In our Restful web service application, we will implement the feature of sending an email with Gmail SMTP Server.
The acronym of SMTP is the Simple Mail Transfer Protocol. Whenever we send a mail to another person’s email id, then there is some protocol through which our email goes through before delivering to the mentioned email id.
Following are the steps required for sending the email via Gmail SMTP.
Step 1: Create a simple Spring Starter Project from STS.
Step 2: Add the Spring Boot Starter Mail dependency in your pom.xml file.
<dependency>
<groupId>
org.springframework.boot
</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
So the final pom.xml file must have following dependencies.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>GmailMessaging</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>GmailMessaging</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Step 3: Configure the Gmail SMTP important properties in the application.propertie
server.port=8083
spring.mail.host=smtp.gmail.com
spring.mail.port=25
spring.mail.username= your gmail id
spring.mail.password= your password
# Other properties
spring.mail.properties.mail.debug=true
spring.mail.properties.mail.transport.protocol=smtp
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.connectiontimeout=5000
spring.mail.properties.mail.smtp.timeout=5000
spring.mail.properties.mail.smtp.writetimeout=5000
# TLS , port 587
spring.mail.properties.mail.smtp.starttls.enable=true
Step 4: Configure the main Spring Boot Application class.
GmailMessagingApplication.java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GmailMessagingApplication {
public static void main(String[] args) {
SpringApplication.run(GmailMessagingApplication.class, args);
}
}
Step 5: Create a Controller class as shown below.
GmailController.java
package com.example.demo.controller;
import org.springframework.beans.factory.annotation.Autowired;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import org.springframework.core.io.ClassPathResource;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GmailController {
@Autowired
private JavaMailSender sender;
@RequestMapping("/hello")
public void Hello() {
System.out.println("ehlloo......");
}
@RequestMapping("/sendMail")
public String sendMail() {
MimeMessage message = sender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message);
try {
helper.setTo("praveenaditya0@gmail.com");
helper.setText("Greetings :)");
helper.setSubject("Mail From Spring Boot");
} catch (MessagingException e) {
e.printStackTrace();
return "Error while sending mail ..";
}
sender.send(message);
return "Mail Sent Success!";
}
@RequestMapping("/sendMailAtt")
public String sendMailAttachment() throws MessagingException {
MimeMessage message = sender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message,true);
try {
helper.setTo("demo@gmail.com");
helper.setText("Greetings :)\n Please find the attached docuemnt for your reference.");
helper.setSubject("Mail From Spring Boot");
ClassPathResource file = new ClassPathResource("document.PNG");
helper.addAttachment("document.PNG", file);
} catch (MessagingException e) {
e.printStackTrace();
return "Error while sending mail ..";
}
sender.send(message);
return "Mail Sent Success!";
}
}
Step 6: Run the Spring Boot Application and hit the endpoint
http://localhost:8083/sendMail
You will get a message as shown below.
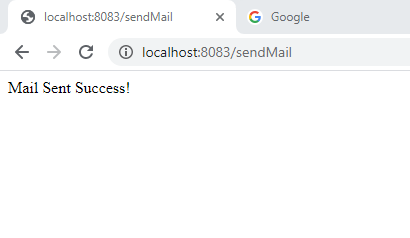
Comments