Null Safety in Kotlin - Kotlin Assignment Help
- Abhay Tiwari
- Dec 13, 2022
- 2 min read
Updated: Feb 15, 2023
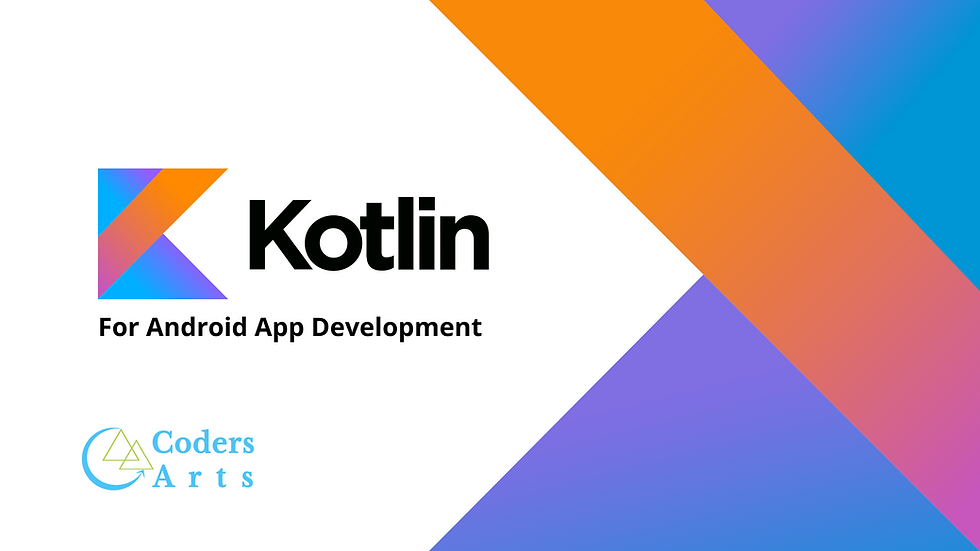
Nullable and Non-Nullable Types in Kotlin
There are two types of references that can hold null (nullable references) and those that can not (non-null references).
A variable of type String can not hold null. If we try to assign null to the variable, it gives a compiler error.
var s1: String = "Abhay"
s1 = null // compilation error
To allow a variable to hold null, we can declare a variable as a nullable string, written String?
var s2: String? = "Abhay"
s2 = null // ok
print(s2)
Kotlin program of non-nullable type:-
fun main(args: Array<String>){
// variable is declared as non-nullable
var s1 : String = "Abhay"
//s1 = null // gives compiler error
print("The length of string s1 is: "+s1.length)
}
Output:
The length of string s1 is: 5
Kotlin program of nullable type-
fun main(args: Array<String>) {
// variable is declared as nullable
var s2: String? = "Abhay"
s2 = null // no compiler error
println(s2.length) // compiler error because string can be null
}
Output:
Error:(8, 15) Kotlin: Only safe (?.) or non-null asserted (!!.) calls are allowed on a nullable receiver of type String?
Checking for null in conditions –
The most common way of checking null reference is using if-else expression. We can explicitly check if variable is null, and handle the two options separately.
Kotlin program of checking null in conditions –
fun main(args: Array<String>) {
// variable declared as nullable
var s: String? = "Abhay"
println(s)
if (s != null) {
println("String of length ${s.length}")
} else {
println("Null string")
}
// assign null
s = null
println(s)
if (s != null) {
println("String of length ${s.length}")
} else {
println("Null String")
}
}
Output:
Abhay
String of length 5
null
Null String
Hope now you are able to understand the nullablity or Null saftey in kotlin in next blog we are going to learn about loops in kotlin.
Thank you
The journey of solving bug and completing project on time in Kotlin can be challenging and lonely. If you need help regarding other sides to Kotlin, we’re here for you!
Comments