Introduction
Welcome to the exciting world of Maps in Java! Maps are an essential part of any programmer, enabling efficient storage and retrieval of key-value pairs. Imagine a map as a magical container that contains a unique key and values, allowing us to retrieve data whenever we needed. With the map, we can store information like Integers, and complex Objects. In this blog, we are going to use and learn the Map and its types.

Understanding
Definition: A map contains values on the basis of a key. i.e. key-value pair also called an entry.
Types: HashMap, TreeMap, LinkedHashMap.
Methods:
put(Key, Value): This method is used to insert data in the map and it will return a null value the first time if you insert the same key with a different value it will return the previous value.
remove(key): It will delete the entry from the map.
get(key): This will return the value associated with a key.
size(): This will return the length of the map.
Types
HashMap: Java HashMap class implements Map interface which allows us to store key and value pair. Hashmap store element without order.
LinkedHashMap: Java LinkedHashMap class that implements Map interface and extends Hashmap class which allows us to store key and value pair in order.
TreeMap: Java TreeMap class that extends SortedMap and that sorted map is implemented in a treemap. It stores elements in sorted order.
Methods that are present in Map classes
keySet(): Return the set of keys.
values(): Return the collection of values.
entrySet(): Return the set of entries. i.e. Key and value pair in the set.
Extra
Map.Entry:
The entry interface that is present in the Map interface will give more access to the map easily. There are different methods present in the Entry interface. This interface we can access only inside the Map interface.
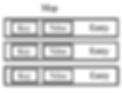
Methods:
getKey(): It will return the key that is present in an entry.
getValue(): It will return the value that is present in an entry.
setValue(value): It will assign the value to a key that is present in an entry.
Implementation
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
public class Main {
public static void main(String[] args) {
Map<Integer, Integer> hashmap = new HashMap<Integer, Integer>();
// To insert the elements
hashmap.put(7, 107);
hashmap.put(2, 102);
hashmap.put(3, 103);
System.out.println("Hashmap Entry :" + hashmap);// Hashmap Entry :{1=101, 2=102, 3=103}
// To remove the elements
hashmap.remove(2);
System.out.println("Hashmap after removing key 2 " + hashmap);// Hashmap after removing key 2 {3=103, 7=107}
// To get the value
int value = hashmap.get(7);
System.out.println("value of 7 key : " + value);// value of 7 key : 107
// To get the size of Map.
System.out.println("Length of map " + hashmap.size()); // Length of map 2
// To insert the element in another map
Map<Integer, Integer> linkedhashmap = new LinkedHashMap<>();
linkedhashmap.putAll(hashmap);
linkedhashmap.put(4, 104);
linkedhashmap.put(5, 105);
// LinkedHashmap
System.out.println("LinkedHashMap follows Order: " + linkedhashmap);// LinkedHashMap follows Order: {3=103,
// 7=107, 4=104, 5=105}
Map<Integer, Integer> treemap = new TreeMap<>();
treemap.putAll(linkedhashmap);
System.out.println("TreeMap follows Natural Order " + treemap);// TreeMap follows Natural Order {3=103, 4=104,
// 5=105, 7=107}
Set<Integer> setkey = treemap.keySet();
// To get all the keys
System.out.println("Key set: " + setkey);// Key set: [3, 4, 5, 7]
// To get all values
Collection<Integer> values = treemap.values();
System.out.println("Values : " + values);// Values : [103, 104, 105, 107]
// To get all entries
Set<Map.Entry<Integer, Integer>> entrySet = treemap.entrySet();
// To print each entry in new line
for (Map.Entry<Integer, Integer> en : entrySet) {
System.out.println(en.getKey() + " -> " + en.getValue());
}
/*
* 3 -> 103
4 -> 104
5 -> 105
7 -> 107
*
*/
}
}
At CodersArts
Expert Assistance: Our team consists of highly skilled Java, and Spring Boot developers who have extensive experience in the field. They are proficient in the latest tools, frameworks, and technologies, ensuring top-notch solutions for your assignments, Projects.
Timely Delivery: We understand the importance of deadlines. Our experts work diligently to deliver your completed assignments, Projects on time, allowing you to submit your work without any worries.
Customized Solutions: We believe in providing personalized solutions that meet your specific requirements. Our experts carefully analyze your assignment or Project and provide tailored solutions to ensure your success.
Affordable Pricing: We offer competitive and affordable pricing to make our services accessible to students, Developers. We understand the financial constraints that students, Developers often face, and our pricing is designed to accommodate their needs.
24/7 Support: Our customer care team is available round the clock to assist you with any queries or concerns you may have. You can reach us via phone, email, or live chat.
Contact CodersArts today for reliable and professional Spring Boot, Spring Security, and Java help. Let our experts take your Java programming skills to the next level!
