Localstorage in React.js - Get Projects Help
- Abhinash Singh
- Jan 4, 2022
- 2 min read
When user logged in a website and leave the website without logging out , when user come in that website again user is logged in already. This is only possible with the help of localstorage. LocalStorage is a web storage object to store the data on the user’s computer locally, which means the stored data is saved across browser sessions and the data stored has no expiration time. We can set ,retrieve and remove data in localstorage
.
Save data in localStorage
import React, { useState } from 'react';
const App = () => {
const [name, setName] = useState('');
const [pwd, setPwd] = useState('');
const handle = () => {
localStorage.setItem('Name', name);
localStorage.setItem('Password', pwd);
};
return (
<div className="App">
<h1>Name of the user:</h1>
<input
placeholder="Name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<h1>Password of the user:</h1>
<input
type="password"
placeholder="Password"
value={pwd}
onChange={(e) => setPwd(e.target.value)}
/>
<div>
<button onClick={handle}>Done</button>
</div>
</div>
)
No library is needed to use Localstorage . Name and password fields are created and after filling the input field localStorage.setItem () is used to store data in localStorage.

Get data from localstorage
import React, { useState } from 'react';
const App = () => {
return (
<div className="App">
{localStorage.getItem('Name') && (
<div>
Name: <p>{localStorage.getItem('Name')}</p>
</div>
)}
{localStorage.getItem('Password') && (
<div>
Password: <p>{localStorage.getItem('Password')}</p>
</div>
)}
<div>
<button onClick={remove}>Remove</button>
</div>
</div>
);
};
export default App;
localStorage.getItem () is used to get data from localStorage.
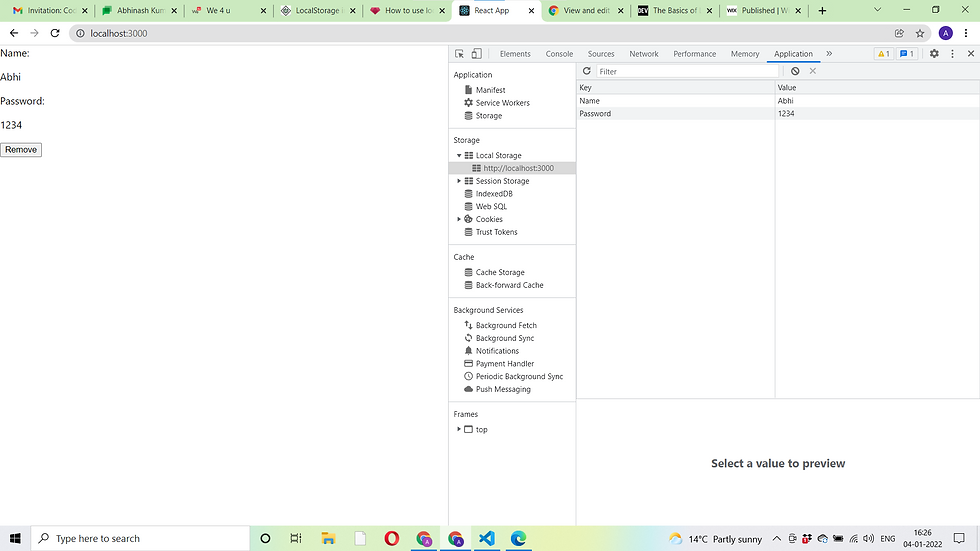
Remove data from localStorage
import React, { useState } from 'react';
const App = () => {
const remove = () => {
localStorage.removeItem('Name');
localStorage.removeItem('Password');
};
return (
<div className="App">
<div>
<button onClick={remove}>Remove</button>
</div>
</div>
);
};
export default App;
When remove button is clicked then remove function is called and to remove data from localStorage.removeItem() then data will be removed.
Are you new to React.js or Just started learning and struggling with react.js project? We have team of react.js developer to help you in react.js project, assignment and for learning react.js at affordable price.
Comments