Kotlin Functions - Kotlin Assignment Help
- Abhay Tiwari
- Jan 25, 2023
- 3 min read
Updated: Feb 15, 2023
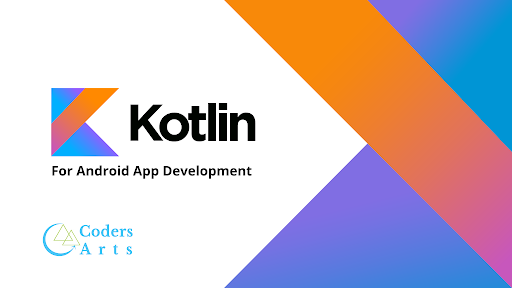
Kotlin is a modern programming language that is fully compatible with Java, and it is known for its expressive syntax, safety features, and improved performance. One of the most powerful features of Kotlin is its functions. Functions are a way to organize and reuse code, and Kotlin provides several features that make functions even more powerful and useful. In this blog, we will explore some of the key features of Kotlin functions with examples.
First, let's take a look at the basic syntax of a Kotlin function:
fun functionName(parameterName: ParameterType): ReturnType {
// function body
}
For example, the following is a simple function that takes two integers as parameters and returns their sum:
fun add(a: Int, b: Int): Int {
return a + b
}
Kotlin also provides support for default parameter values. This means that if a value is not provided for a parameter when the function is called, a default value will be used instead. For example, the following function has a default value for the "b" parameter:
fun add(a: Int, b: Int = 0): Int {
return a + b
}
Kotlin also provides support for named arguments. This means that when calling a function, you can specify the names of the parameters along with their values. This can make code more readable and understandable. For example, the following code calls the "add" function with named arguments:
val result = add(a = 2, b = 3)
Kotlin also provides support for higher-order functions, which are functions that take other functions as arguments or return them as results. This allows for even greater flexibility and code reuse. For example, the following code defines a higher-order function that takes a function as an argument and calls it:
fun execute(function: (Int) -> Unit) {
function(5)
}
Here, the execute function takes a function as an argument, which takes an int and returns nothing.
Kotlin also provides support for lambda expressions, which are anonymous functions that can be used to create short, inline functions. These are especially useful when you need to pass a simple function as an argument to another function. Lambda expressions are defined using the "->" operator, and they can be passed as arguments to other functions, or assigned to variables.
val square: (Int) -> Int = {x -> x*x}
Here, we have defined a lambda expression which takes an int as input and returns its square.
In addition to these features, Kotlin also provides support for function types, which are types that represent functions. Function types are defined using the "FunctionN" syntax, where N is the number of arguments the function takes. For example, a function that takes two arguments and returns a value is of type "Function2<A, B, R>", where A and B are the types of the arguments, and R is the type of the return value.
Copy code
val add: (Int, Int) -> Int = {a, b -> a + b}
Here, we have defined a variable 'add' which is of type Function2 and takes two integers as input and returns their sum.
Kotlin also provides support for extension functions, which allow you to add new functions to existing classes without having to inherit from them. Extension functions are defined using the "extension" keyword
Hope you understand the functions and syntax of kotlin in the next blog we are going learn Kotlin - Control Flow in kotlin. Thank you The journey of solving bugs and completing projects on time in Kotlin can be challenging and lonely. If you need help regarding other sides of Kotlin, we’re here for you! Drop an email to us at contact@codersarts.com with the Project title, deadline, and requirement files. Our email team will revert back promptly to get started on the work.
Comentarios