FastAPI Boilerplate: A Quick Start Guide for Building Web Apps
- Codersarts
- Feb 5, 2024
- 2 min read
Updated: Feb 6, 2024
Creating a boilerplate code for a FastAPI app involves setting up the basic structure and dependencies needed to start building your application.
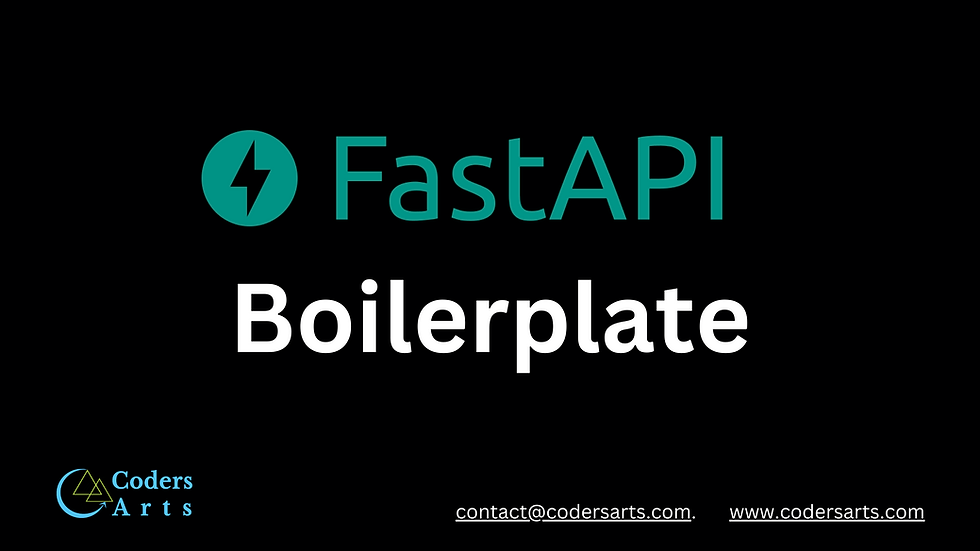
Here are step-by-step instructions to create a simple FastAPI boilerplate code:
Install FastAPI and Uvicorn:
Open your terminal or command prompt and run the following commands to install FastAPI and Uvicorn:
pip install fastapi
pip install uvicorn
Create a Main FastAPI App File:
Create a new Python file (e.g., main.py) for your FastAPI application. This file will contain the main FastAPI application code.
# main.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"message": "Hello, FastAPI!"}
Create a Requirements File:
Create a requirements.txt file to list the dependencies of your FastAPI app. This is useful for others to replicate your environment.
fastapi==0.70.0
uvicorn==0.15.0
You can generate this file by running:
pip freeze > requirements.txt
Create a Dockerfile (Optional):
If you want to containerize your FastAPI app using Docker, create a Dockerfile in the same directory as your main FastAPI app file. Here's a simple example:
# Dockerfile
FROM tiangolo/uvicorn-gunicorn-fastapi:python3.9
COPY ./app /app
This assumes that your FastAPI app is in a directory named app.
Run Your FastAPI App:
Open the terminal and run your FastAPI app using Uvicorn:
uvicorn main:app --reload
Access your app in the browser at http://127.0.0.1:8000 or explore the interactive documentation at http://127.0.0.1:8000/docs
This simple setup provides a starting point for your FastAPI app. You can then expand it by adding more routes, dependencies, and features as needed for your specific application. Additionally, you can explore more advanced configurations, such as database connections, authentication, and asynchronous programming, based on your project requirements.
Project Generation - Template
Full Stack FastAPI PostgreSQL
Full Stack FastAPI Couchbase
Machine Learning models with spaCy and FastAPI¶
Comentários