AngularJs Basics: AngularJs Directives
- naveen kumar
- Sep 17, 2020
- 2 min read

In AngularJS directives are used to extend the use of HTML. They are special attributes starting with ng-prefix. Below the list of directives:
ng-app
ng-init
ng-model
ng-repeat
ng-bind
ng-show
ng-controller
ng-disabled
ng-if
ng-click
etc.
ng-app directive
The ng-app directive starts an AngularJS Application and it used as the root element. It automatically initializes the application when the web page containing AngularJS Application is loaded.
it define using div:
<div ng-app = "">
...
</div>
ng-init directive
The ng-init directive initializes an AngularJS Application data.
it assign value to the variables.
<div ng-app="" ng-init="firstName='naveen'">
<p>The name is <span ng-bind="firstName"></span></p>
</div>
or in collection form
<div ng-app = "" ng-init = "countries = [{locale:'en-US',name:'United States'},
{locale:'en-GB',name:'United Kingdom'}, {locale:'en-FR',name:'France'}]">
...
</div>
Example:
<!DOCTYPE html>
<html >
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script>
</head>
<body >
<div ng-app ng-init="greet='Hello World!'; amount= 1000; myArr = [100, 200]; person = { firstName:'naveen', lastName :'kumar'}">
{{amount}} <br />
{{myArr[1]}} <br />
{{person.firstName}}
</div>
</body>
</html>
ng-model directive
The ng-model directive defines the model/variable to be used in AngularJS Application. In the following example, we define a model named name.
<div ng-app = "">
...
<p>Enter your Name: <input type = "text" ng-model = "name"></p>
</div>
Example:
<!DOCTYPE html>
<html >
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script>
</head>
<body ng-app>
<input type="text" ng-model="name" />
<div>
Hello {{name}}
</div>
</body>
</html>
ng-repeat directive
The ng-repeat directive repeats HTML elements for each item in a collection. In the following example, we iterate over the array of countries
Example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script>
</head>
<body ng-app="" ng-init="students=['student1','Student2','student3']">
<ol>
<li ng-repeat="name in students">
{{name}}
</li>
</ol>
<div ng-repeat="name in students">
{{name}}
</div>
</body>
</html>
ng-bind directive
Replaces the value of HTML control with the value of specified AngularJS expression.
Example:
<!DOCTYPE html>
<html >
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script>
</head>
<body ng-app="">
<div>
1 + 2 = <span ng-bind="1 + 2"></span> <br />
Enter your name: <input type="text" ng-model="name" /><br />
Hello <span ng-bind="name"></span>
</div>
</body>
</html>
Output:

ng-disabled directive:
Sets the disable attribute on the HTML element if specified expression evaluates to true.
Example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script>
</head>
<body ng-app ng-init="checked=true" >
Click Me: <input type="checkbox" ng-model="checked" /> <br />
<div>
New: <input ng-if="checked" type="text" />
</div>
<div>
Read-only: <input ng-readonly="checked" type="text" value="This is read-only." />
</div>
<div>
Disabled: <input ng-disabled="checked" type="text" value="This is disabled." />
</div>
</body>
</html>
Output:
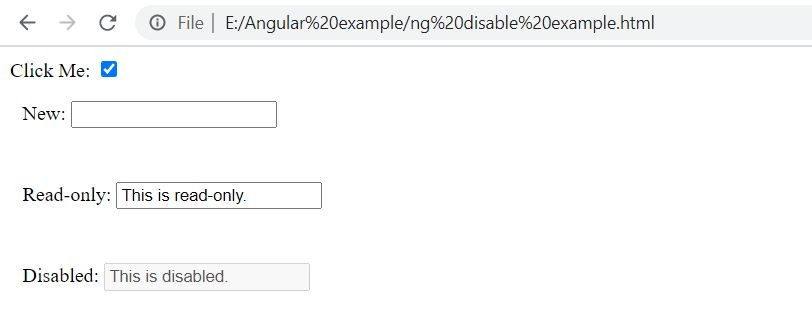
Get instant help at here:
contact@codersarts.com
Comments